一、IO流概述
1.原理
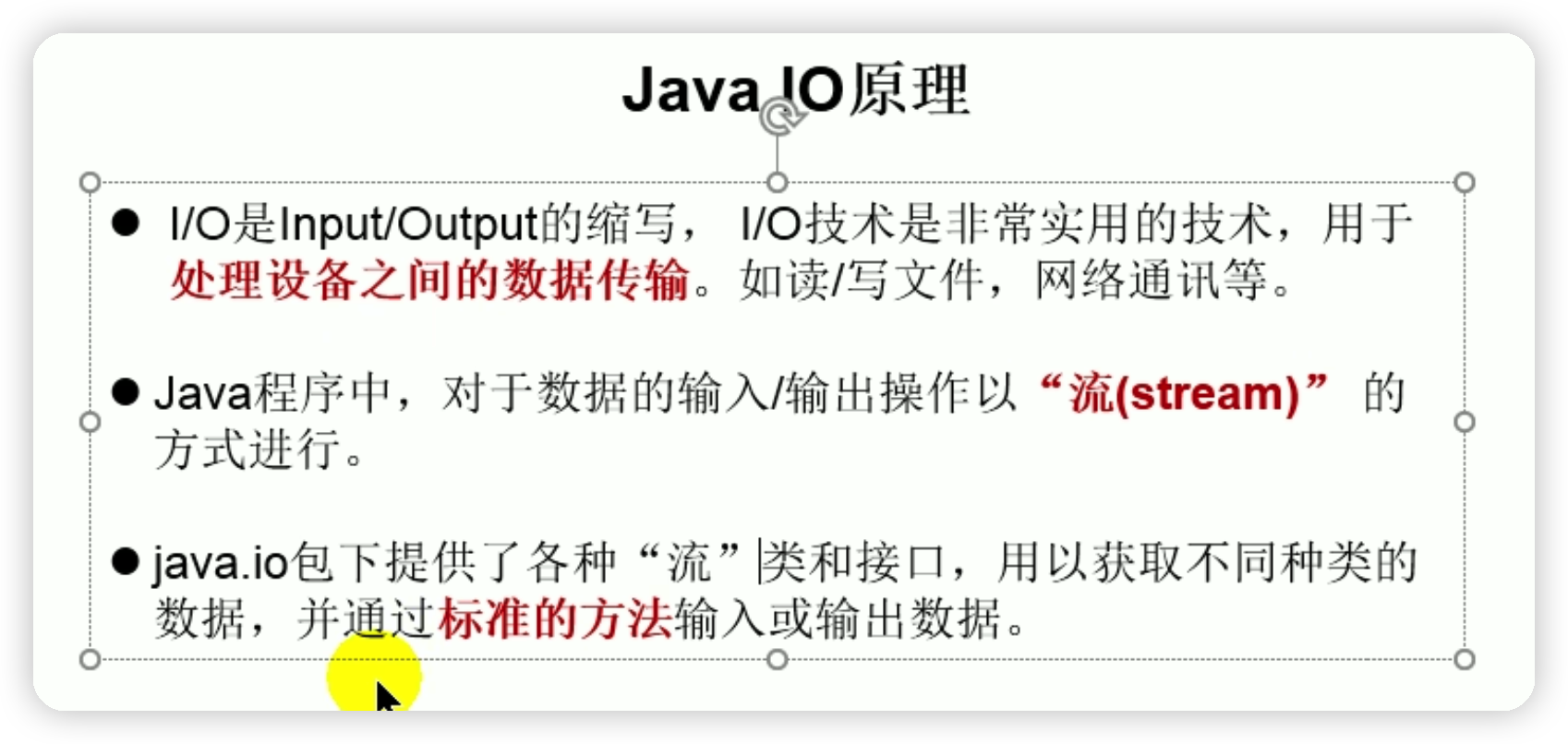
2.流的分类
3.流的体系,蓝底框为重点掌握的
二、IO流操作
1.节点流-字符流
(1).FileReader读入数据的基本操作
点击查看代码
package com.Tang.io;
import org.junit.Test;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
public class IOTest {
@Test
public void test() {
FileReader fr = null;
//为了保证流资源一定可以执行关闭操作,需要使用try-catch-finally
//读入的文件一定要存在,否则就会报FileNotFoundException。
try {
//将Hello工程下的hello.txt文件内容读入程序中,并输出到控制台
//1.实例化File类对象,指明要操作的文件
File file = new File(\"hello.txt\");
//2.提供具体的流
fr = new FileReader(file);
//3.数据的读入
//read():返回读入的一个字符,如果达到文件末尾,返回-1;否则返回字符的Ascall值
int data = fr.read();
while(data != -1){
System.out.print((char)data);//读取文件第一个字符
data = fr.read();//读取文件下一个字符
}
} catch (IOException e) {
e.printStackTrace();
} finally {
//4.流的关闭操作
try {
if(fr != null)
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
运行结果图
①.FileReader对read()操作升级:使用read的重载方法
代码中for循环处如果写为i < cubf.length会出现一下问题
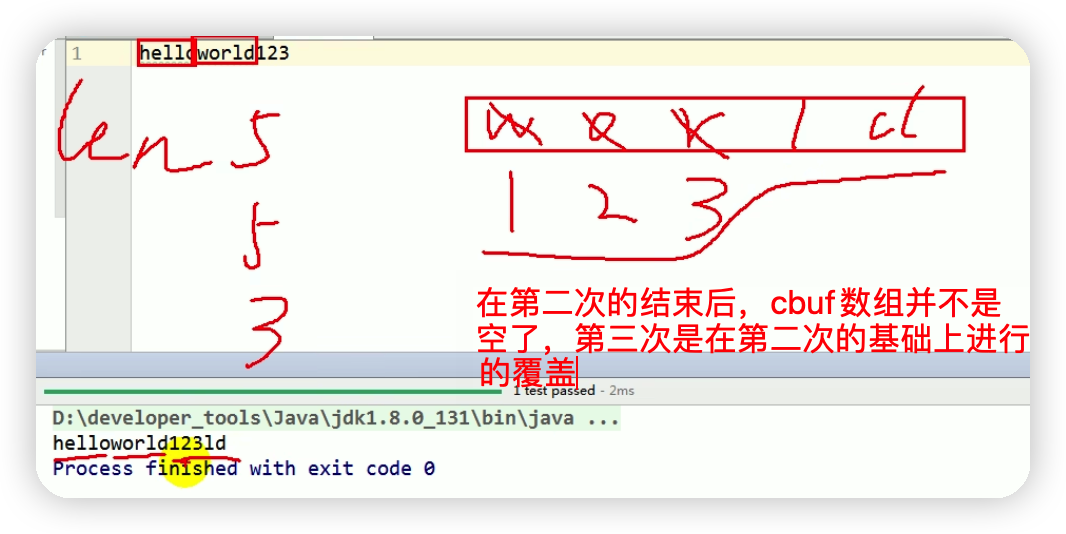
点击查看代码
//对read()操作升级:使用read的重载方法
@Test
public void test1(){
FileReader fr = null;
try {
//1.File类的实例化
File file = new File(\"hello.txt\");
//2.FileReader流的实例化
fr = new FileReader(file);
//3.读入的操作
//read(char[] cbuf):返回每次读入cbuf数组中的字符的个数,当读到文件末尾时返回-1
char[] cbuf = new char[5];//相当于一个容量池,每次能从文件能读出的最大字符数
int len;
while((len = fr.read(cbuf) )!= -1){
//方式一:
//错误写法
// for (int i = 0; i <cbuf.length ; i++) {
// System.out.print(cbuf[i]);
// }
//正确写法:每次读到几个字符就输出几个
// for (int i = 0; i <len; i++) {
// System.out.print(cbuf[i]);
// }
//方式二:
// 将数组转化为字符,因为每一次读到的字符都是在上一次数组上的覆盖
//因此每次只需取出从数组开始位置到所能读到的字符的即可结束
String str = new String(cbuf,0,len);
System.out.print(str);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fr != null){
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
// fr.read(cbuf);
}
运行结果图
(2)FileWriter写出数据的操作
点击查看代码
/*
1.输出操作,对应的File可以不存在,并不会报异常
2.
File对应的硬盘中的文件如果不存在:在输出的过程中,会自动创建此文件
File对应的硬盘中的文件如果存在:
如果流使用的构造器是FileWriter(file,false) / FileWriter(false):对原有文件的覆盖
如果流使用的构造器是FileWriter(file,true) /:不会对原有文件覆盖,而是在原有文件基础上追加内容
*/
@Test
public void test2() {
FileWriter fw = null;
try {
//1.提供File类的对象,指明写出到的文件
File file = new File(\"hello1.txt\");
//2.提供FileWriter的对象,用于数据的写出
fw = new FileWriter(file);
//3.写出的操作
fw.write(\"I have a dream\\n\");
fw.write(\"you need to hava a dream\");
} catch (IOException e) {
e.printStackTrace();
} finally {
//4.流资源的关闭
if(fw != null){
try {
fw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
运行结果图
(3).使用FileReader和FileWriter实现文本文件的复制
①一开始就按下方图片的代码去写,然后选中除关闭流的以外的代码按快捷键ctrl + alt + t生成 try - catch - finally然后将关闭流的代码放入finally中并单独生成 try - catch
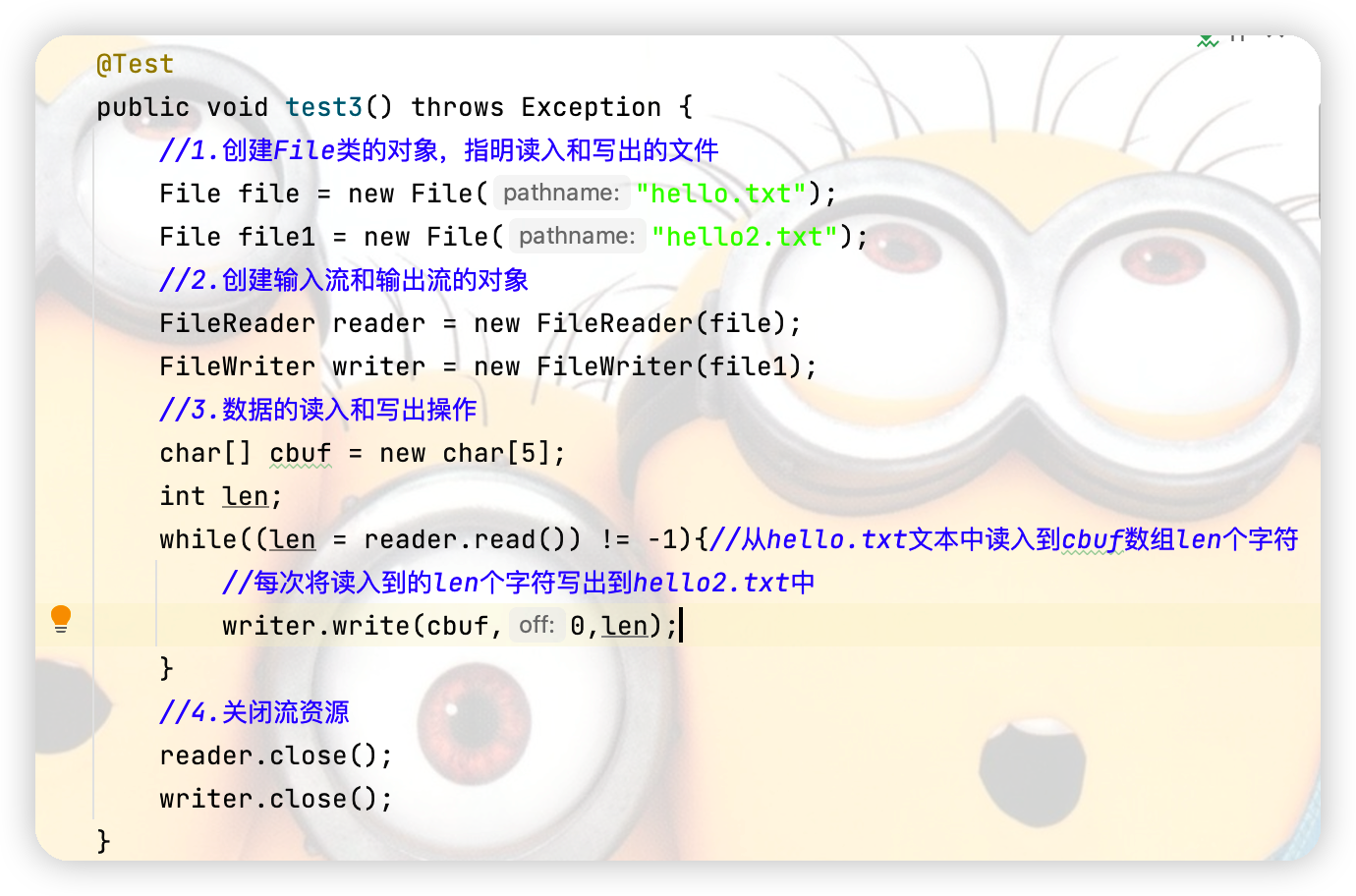
点击查看代码
@Test
public void test3(){
FileReader fr = null;
FileWriter fw = null;
try {
//1.创建File类的对象,指明读入和写出的文件
File file = new File(\"hello.txt\");
File file1 = new File(\"hello2.txt\");
//2.创建输入流和输出流的对象
fr = new FileReader(file);
fw = new FileWriter(file1);
//3.数据的读入和写出操作
char[] cbuf = new char[5];
int len;
while((len = fr.read(cbuf)) != -1){//从hello.txt文本中读入到cbuf数组len个字符
//每次将读入到的len个字符写出到hello2.txt中
fw.write(cbuf,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
//4.关闭流资源
try {
if(fw !=null)
fw.close();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if(fr != null)
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
运行结果图
注意:字符流不能处理图片文件的测试
2.节点流-字节流
(1)FileInputStream的使用
①字节流处理文本文件
点击查看代码
/*
结论:
1.对于文本文件(.txt , .java , .c , .cpp)使用字符流处理
2.对于非文本文件(.jpg, .mp3, .mp4, .doc, .ppt ......)使用字节流处理
*/
@Test
public void test4(){
//使用字节流FileInputStream处理文本文件,可能出现乱码
FileInputStream fis = null;
try {
//1.造文件
File file = new File(\"hello.txt\");
//2.造流
fis = new FileInputStream(file);
//3.读数据
byte[] bytes = new byte[5];
int len;//记录每次读取的字节的个数
while((len=fis.read(bytes)) != -1){
String str = new String(bytes,0,len);
System.out.println(str);
}
} catch (IOException e) {
e.printStackTrace();
} finally {//4.关闭数据
try {
if(fis != null)
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
②字节流处理非文本文件
点击查看代码
//实现对图片的复制
@Test
public void test5() {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
File file = new File(\"QQ20210927-0.jpg\");
File file1 = new File(\"QQ20210927-1.jpg\");
fis = new FileInputStream(file);
fos = new FileOutputStream(file1);
byte[] bytes = new byte[5];
int len ;
while((len = fis.read(bytes))!=-1){
fos.write(bytes,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fis != null){
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fos != null){
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
运行结果图
(2)FileInputStream和FileOutputStream复制文件的方法测试
点击查看代码
//指定路径下的文件复制
public void copyFile(String srcPath,String destPath){
FileInputStream fis = null;
FileOutputStream fos = null;
try {
File file = new File(srcPath);
File file1 = new File(destPath);
fis = new FileInputStream(file);
fos = new FileOutputStream(file1);
byte[] bytes = new byte[1024];
int len ;
while((len = fis.read(bytes))!=-1){
fos.write(bytes,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fis != null){
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fos != null){
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void test6(){
long start = System.currentTimeMillis();
String srcPath = \"/Users/twq/Downloads/01.mp4\";
String destPath = \"/Users/twq/Downloads/03.mp4\";
copyFile(srcPath,destPath);
long end = System.currentTimeMillis();
System.out.println(\"复制操作花费的时间为:\"+(end - start));
}
运行结果图
3.缓冲流-字节流
(1)实现非文本文件的复制
点击查看代码
/*
实现非文本文件的复制
*/
@Test
public void test7() {
FileInputStream fis = null;
FileOutputStream fos = null;
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
//1.造文件
File file = new File(\"QQ20210927-0.jpg\");
File file1 = new File(\"QQ20210927-2.jpg\");
//2.造流
//2.1造节点流
fis = new FileInputStream(file);
fos = new FileOutputStream(file1);
//2.2造缓冲流:处理流是包装在节点流之上的
bis = new BufferedInputStream(fis);
bos = new BufferedOutputStream(fos);
//3.复制的细节:读取、写入
byte[] bytes = new byte[1024];
int len;
while((len = bis.read(bytes))!= -1){
bos.write(bytes,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(bis != null){
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(bos != null){
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
//4.资源关闭
//要求:先关闭外层的流,在关闭内层的流
//关闭外层流的同时,内层流也会自动进行关闭,关于内层流的关闭我们可以省略
// fos.close();
// fis.close();
}
运行结果图
(2)缓冲流相较于节点流的优势
点击查看代码
/*
实现非文本文件的复制
*/
@Test
public void test7() {
long start = System.currentTimeMillis();
String srcPath = \"/Users/twq/Downloads/01.mp4\";
String destPath = \"/Users/twq/Downloads/02.mp4\";
copyFileBuffer(srcPath,destPath);
long end = System.currentTimeMillis();
System.out.println(\"缓冲流复制操作花费的时间为:\"+(end - start));
}
//指定路径下的文件复制
public void copyFileBuffer(String srcPath,String destPath){
FileInputStream fis = null;
FileOutputStream fos = null;
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
//1.造文件
File file = new File(srcPath);
File file1 = new File(destPath);
//2.造流
//2.1造节点流
fis = new FileInputStream(file);
fos = new FileOutputStream(file1);
//2.2造缓冲流:处理流是包装在节点流之上的
bis = new BufferedInputStream(fis);
bos = new BufferedOutputStream(fos);
//3.复制的细节:读取、写入
byte[] bytes = new byte[1024];
int len;
while((len = bis.read(bytes))!= -1){
bos.write(bytes,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(bis != null){
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(bos != null){
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
//4.资源关闭
//要求:先关闭外层的流,在关闭内层的流
//关闭外层流的同时,内层流也会自动进行关闭,关于内层流的关闭我们可以省略
// fos.close();
// fis.close();
}
运行结果图
缓冲流能提高读写速度的原因:内部提供了一个缓冲区
4.缓冲流-字符流
使用BufferReader和BufferWriter实现文本文件的复制
点击查看代码
//使用BufferReader和BufferWriter实现文本文件的复制
@Test
public void test8() {
BufferedReader br = null;
BufferedWriter bw = null;
try {
//创建文件和相应的流
br = new BufferedReader(new FileReader(new File(\"hello.txt\")));
bw = new BufferedWriter(new FileWriter(new File(\"hello3.txt\")));
//读
char[] chars = new char[1024];
int len ;
while((len = br.read(chars))!= -1){
bw.write(chars,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(br != null){
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(bw != null){
try {
bw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
5.图片的加解密
相同的代码在运行一次就可以进行解密,主要是因为两次相同的异或之后可以得到运来的数据
6.转换流
(1)InputStreamReader和OutputStreamWriter都属于字符流,作用都是提供字节流与字符流之间的转换
①InputStreamReader:将一个字节的输入流转换为字符的输入流
点击查看代码
@Test
public void test() {
InputStreamReader isr = null;
try {
FileInputStream fis = new FileInputStream(\"hello.txt\");
// InputStreamReader isr = new InputStreamReader(fis);//使用系统默认的字符集
//参数2指明了字符集,具体使用那个字符集,取决于文件hello.txt保存时使用的字符集
isr = new InputStreamReader(fis,\"UTF-8\");
char[] chars = new char[20];
int len ;
while((len = isr.read(chars))!= -1){
String s = new String(chars, 0, len);
System.out.println(s);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(isr != null){
try {
isr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
运行结果图:
②OutputStreamWriter:将一个字符的输出流转为字节的输出流
(2)综合使用InputStreamReader和OutputStreamWriter
点击查看代码
@Test
public void test1(){
InputStreamReader isr = null;
OutputStreamWriter osw = null;
try {
File file = new File(\"hello.txt\");
File file1 = new File(\"hello4.txt\");
FileInputStream fis = new FileInputStream(file);
FileOutputStream fos = new FileOutputStream(file1);
isr = new InputStreamReader(fis);
osw = new OutputStreamWriter(fos,\"gbk\");
char[] chars = new char[20];
int len;
while((len = isr.read(chars)) != -1){
osw.write(chars,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(isr != null){
try {
isr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(osw != null){
try {
osw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
运行结果图
7.数据流
(1)DataInputStream 和 DataOutputStream作用:拥有读取或写出基本数据类型的变量或字符串
点击查看代码
将文件中存储的基本数据类型变量和字符串读取到内存中,保存在变量中
注意点:读取不同类型的数据要与当初写入文件时,保存的数据的顺序一致!
*/
@Test
public void test3() {
DataInputStream dis = null;
try {
dis = new DataInputStream(new FileInputStream(\"data.txt\"));
String name = dis.readUTF();
int age = dis.readInt();
boolean isMale = dis.readBoolean();
System.out.println(\"name = \" + name);
System.out.println(\"age = \" + age);
System.out.println(\"isMale = \" + isMale);
} catch (IOException e) {
e.printStackTrace();
} finally {
if(dis != null){
try {
dis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void test2() {
DataOutputStream dos = null;
try {
dos = new DataOutputStream(new FileOutputStream(\"data.txt\"));
dos.writeUTF(\"唐昊\");
dos.flush();//刷新操作,将内存中的数据写入文件
dos.writeInt(23);
dos.flush();
dos.writeBoolean(true);
dos.flush();
} catch (IOException e) {
e.printStackTrace();
} finally {
if(dos != null){
try {
dos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
运行结果图
注意:需要先运行向文件里写的操作,才能继续运行读的操作,并且读的顺序必须与写的顺序一致,否则就会报EOFException异常
8.对象流
(1)ObjectInputStream和ObjectOutputStream:用于存储和读取基本数据类型数据或对象的处理流
①序列化与反序列化
点击查看代码
/*
反序列化:将磁盘文件的对象还原为内存中的一个Java对象
使用ObjectInputStream来实现
*/
@Test
public void test6(){
ObjectInputStream ois = null;
try {
ois = new ObjectInputStream(new FileInputStream(\"Object.txt\"));
Object o = ois.readObject();
String str = (String)o;
Person p =(Person) ois.readObject();
System.out.println(str);
System.out.println(p);
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
} finally {
if(ois != null){
try {
ois.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
/*
序列化过程:将内存中的Java对象保存到磁盘中或通过网络传输出去
使用ObjectOutputStream实现
*/
@Test
public void test5(){
ObjectOutputStream oos = null;
try {
oos = new ObjectOutputStream(new FileOutputStream(\"Object.txt\"));
oos.writeObject(new String(\"北京天安门\"));
oos.flush();//刷新操作
//要想一个Java对象时可序列化的,需要满足相应的要求,见Person.java
oos.writeObject(new Person(\"王名\",23));
oos.flush();
} catch (IOException e) {
e.printStackTrace();
} finally {
if(oos != null){
try {
oos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
②自定义类的序列化与反序列化
Person类代码如下
点击查看代码
package com.Tang.io;
import java.io.Serializable;
/*
Person需要满足如下的要求,方可序列化
1.需要实现接口:Seriallizabe
2.当前类提供一个全局常量:serialVersionUID
3.除了当前Person类需要实现Serializable接口之外,还必须保证其内部所有属性也必须是可序列化的(默认情况下:基本数据类型可序列化
*/
public class Person implements Serializable {
public static final long serialVersionUID = 3476465475L;
private String name;
private int age;
public Person() {
}
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
@Override
public String toString() {
return \"Person{\" +
\"name=\'\" + name + \'\\\'\' +
\", age=\" + age +
\'}\';
}
}
运行结果图:
注:代码的运行得先运行序列化的代码然后再运行反序列化的代码
③public static final long serialVersionUID :这个id如果没有写的话,就进行的序列化操作(没有进行反序列化),然后对类进行一些修改之后,在进行反序列化就会报错,起初定义好序列化id是为了反序列化能根据此id进行无差错的反序列化
9.RandomAccessFile的使用
(1)实现非文本文件的复制
点击查看代码
/*
RandomAccessFile的使用
1.RandomAccessFile直接继承与Java.lang.object类,实现了DataInput和DataOutput接口
2.RandomAccessFile既可以作为一个输入流,又可以作为一个输出流
*/
@Test
public void test7(){
RandomAccessFile raf = null;
RandomAccessFile rw = null;
try {
raf = new RandomAccessFile(new File(\"hello.txt\"), \"r\");
rw = new RandomAccessFile(new File(\"hello1.txt\"), \"rw\");
byte[] bytes = new byte[1024];
int len;
while((len = raf.read(bytes))!= -1){
rw.write(bytes,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(raf != null){
try {
raf.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(rw != null){
try {
rw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
(2)单独作为输出流时
点击查看代码
/*
如果RandomAccessFile作为输出流时,写出到的文件如果不存在,则在执行过程中自动创建
如果写到的文件存在,则会对原有文件从头开始覆盖
*/
@Test
public void test8(){
RandomAccessFile raf = null;
try {
raf = new RandomAccessFile(new File(\"hello.txt\"),\"rw\");
raf.write(\"xyz\".getBytes(StandardCharsets.UTF_8));
} catch (IOException e) {
e.printStackTrace();
} finally {
if(raf != null){
try {
raf.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
运行之前hello.txt文件里的内容如下图
上述代码运行之后hello.txt 中的内容如下
(3)实现在文件指定位置插入数据
点击查看代码
@Test
public void test8(){
RandomAccessFile raf = null;
try {
raf = new RandomAccessFile(new File(\"hello.txt\"),\"rw\");
raf.seek(3);//将指针调到角标为3的位置
//保存指针3后面的所有数据到StringBuilder中
StringBuilder builder = new StringBuilder((int) new File(\"hello.txt\").length());
byte[] bytes = new byte[20];
int len;
while((len = raf.read(bytes))!= -1){
builder.append(new String(bytes,0,len));
}
//调回指针写入\"xyz\"
raf.seek(3);
raf.write(\"xyz\".getBytes());
//将StringBuilder 中的数据写入到文件中
raf.write(builder.toString().getBytes(StandardCharsets.UTF_8));
} catch (IOException e) {
e.printStackTrace();
} finally {
if(raf != null){
try {
raf.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
代码运行前hello.txt中的内容如下
运行代码在文件内容角标为3的位置插入xyz之后结果如下
来源:https://www.cnblogs.com/twq46/p/16470524.html
本站部分图文来源于网络,如有侵权请联系删除。